Our Courses
Join Our Classes
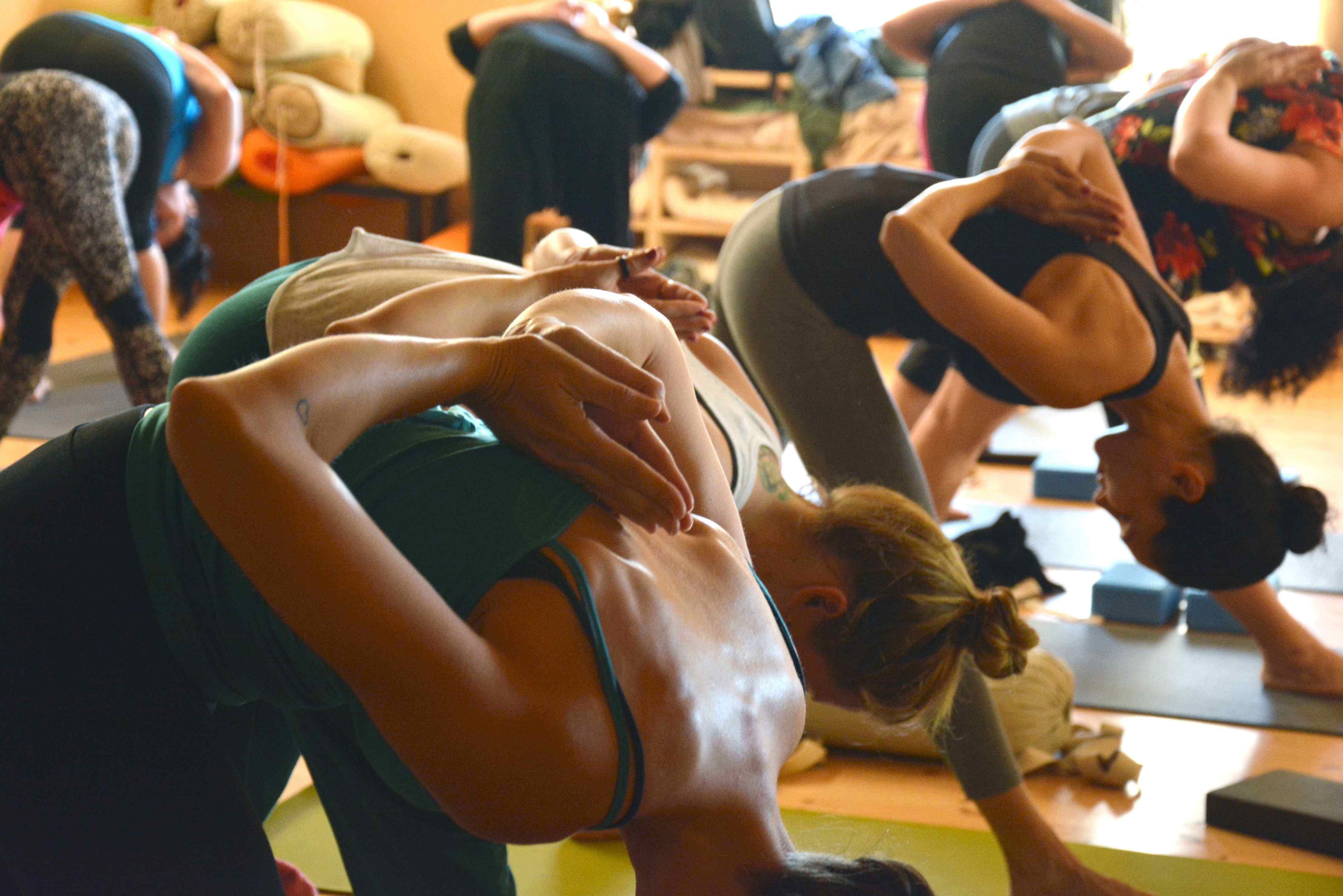
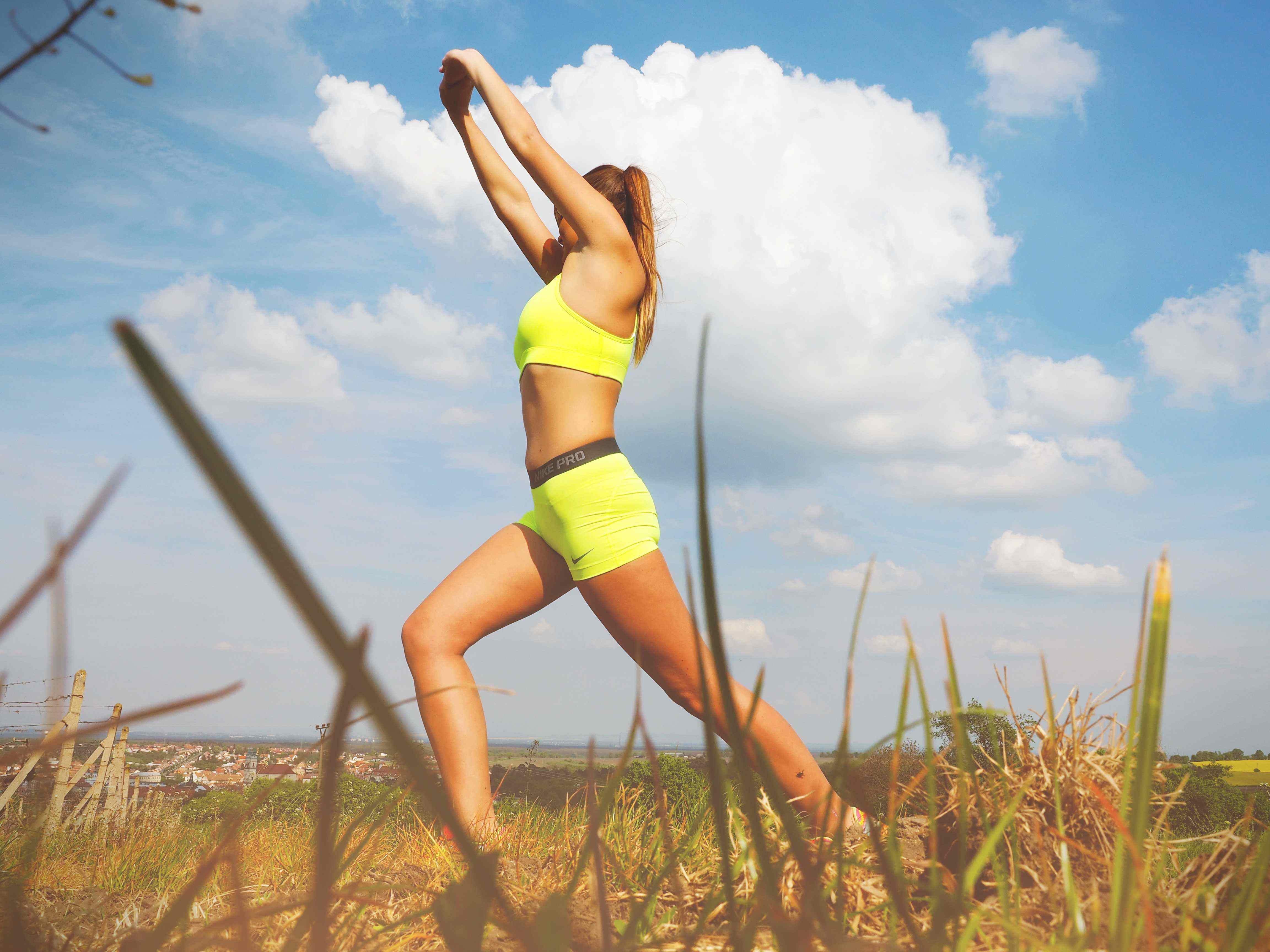
$ 30
Florence Pugh
Group Lessons

7:00 to 8:00 am

25 Peoples
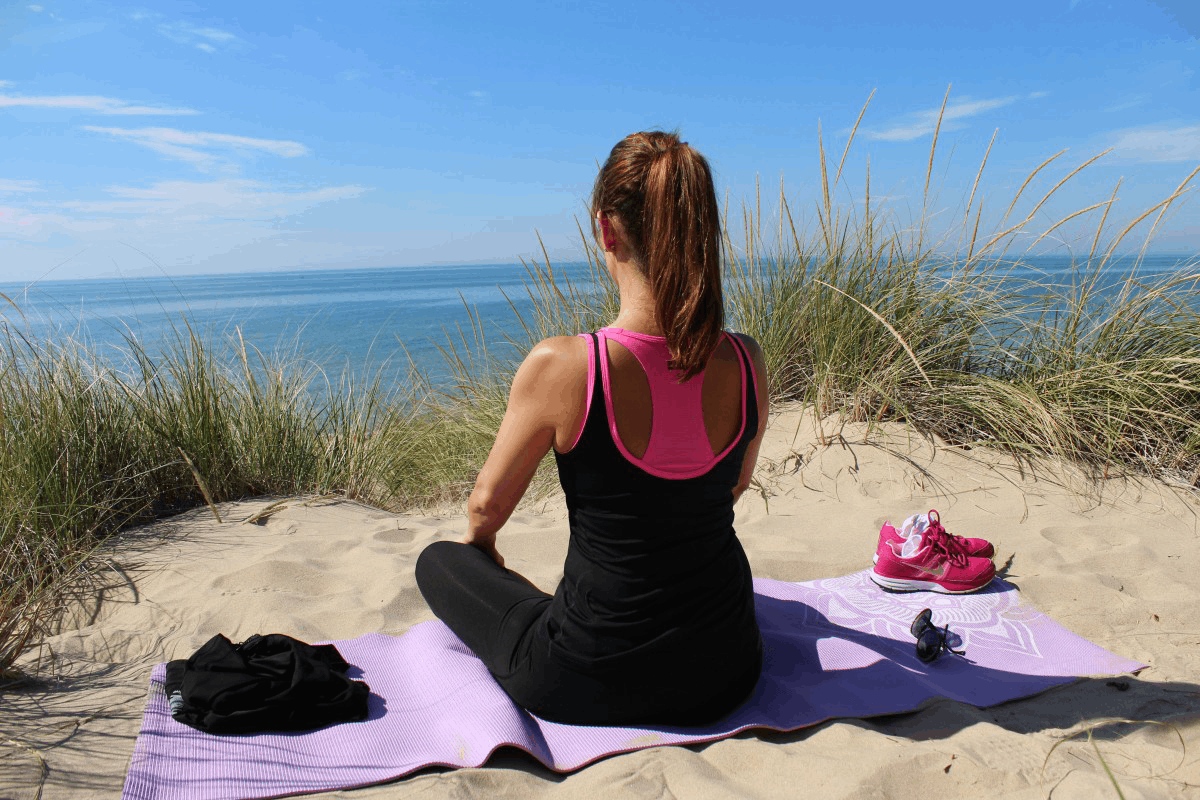
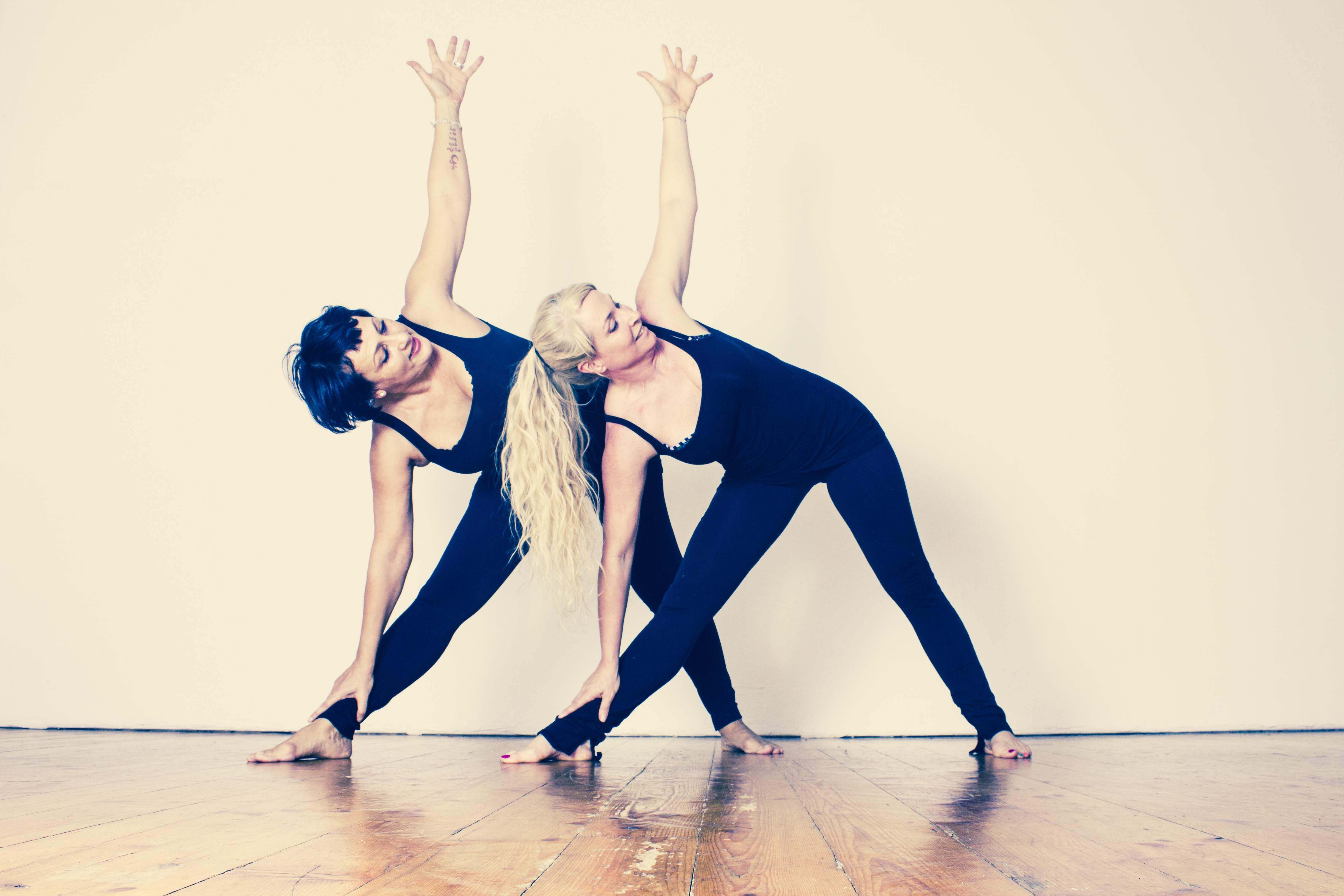
$ 30
Florence Pugh
Group Lessons

7:00 to 8:00 am

25 Peoples
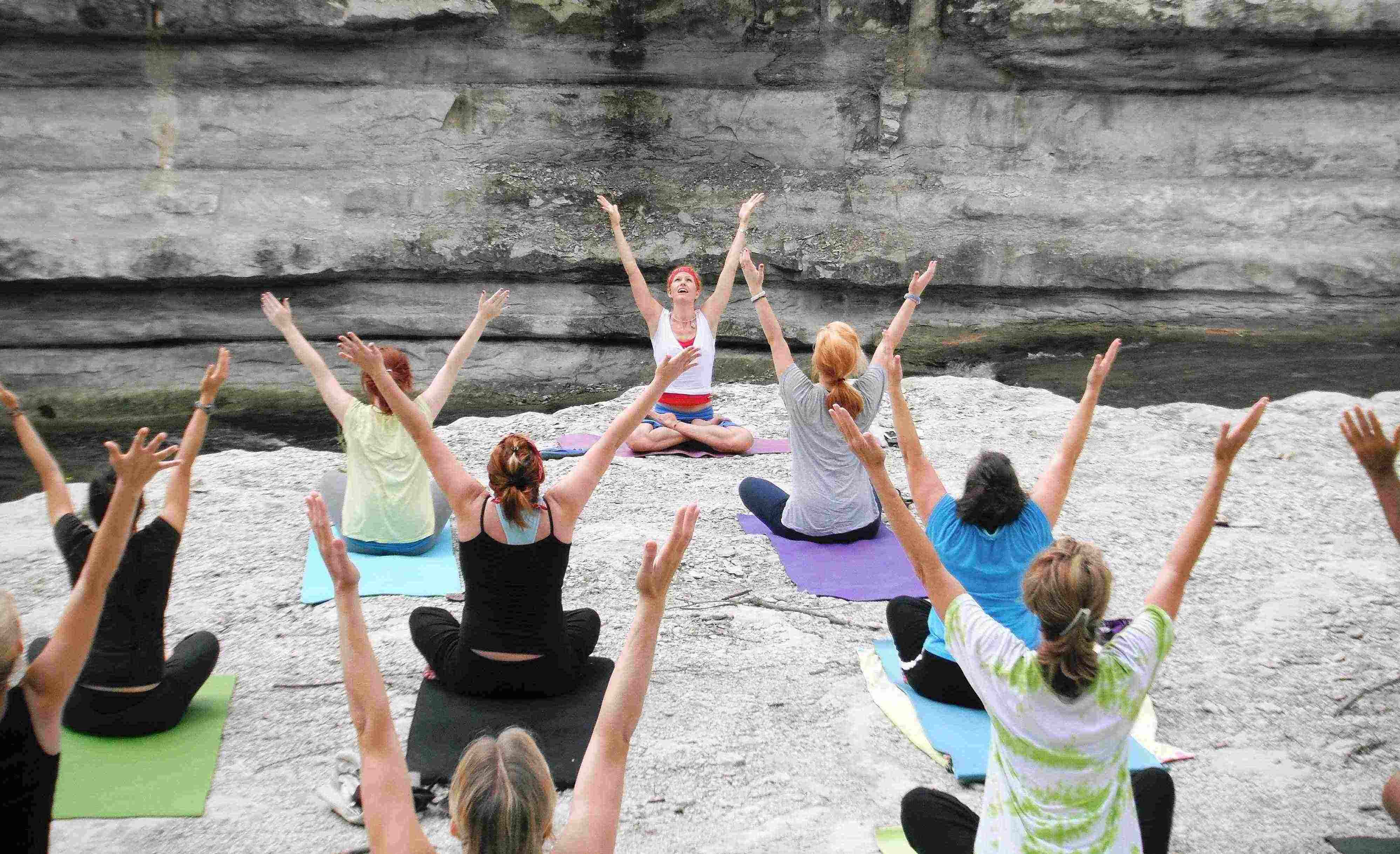
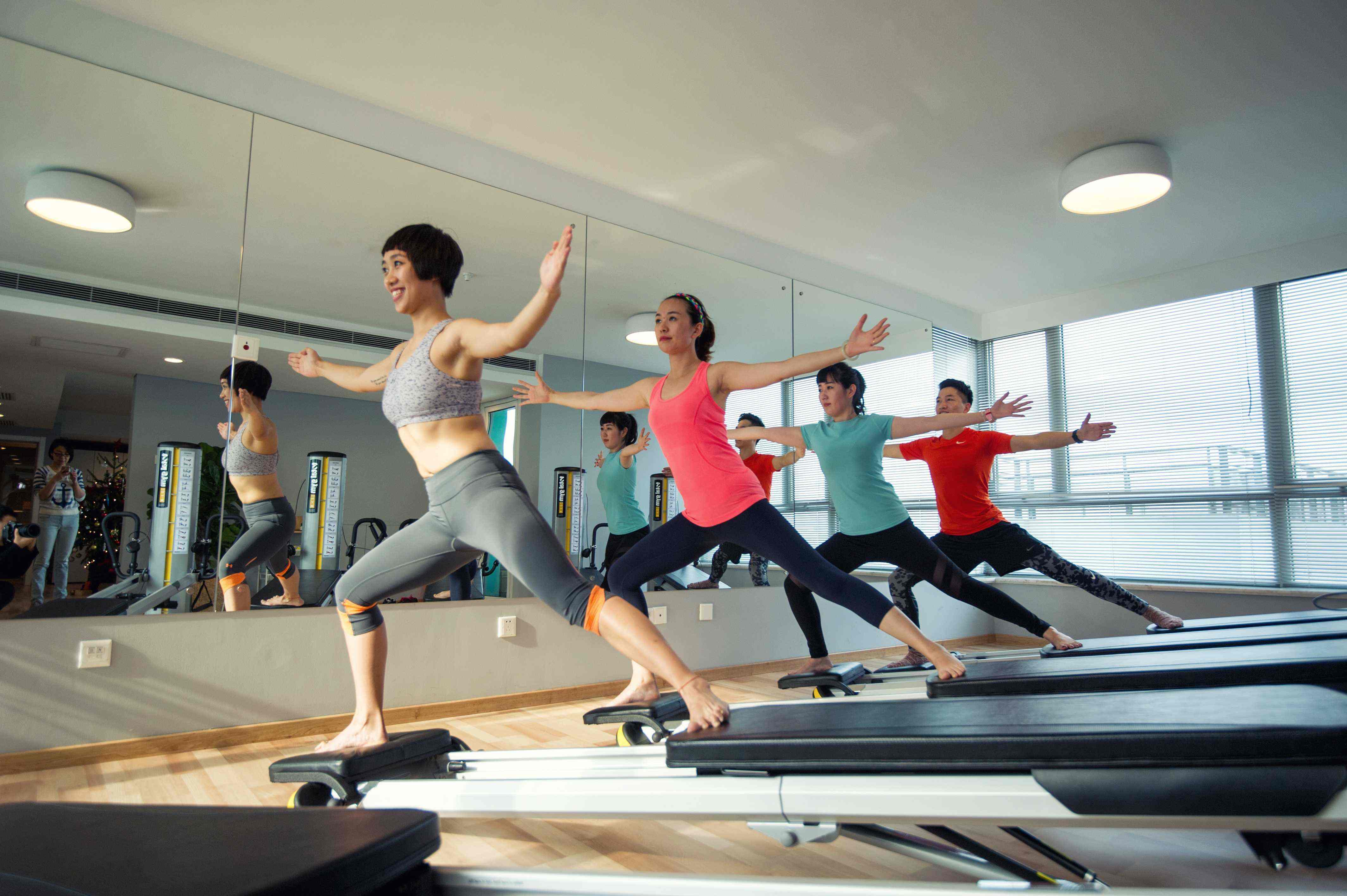
$ 30
Florence Pugh
Group Lessons

7:00 to 8:00 am

25 Peoples
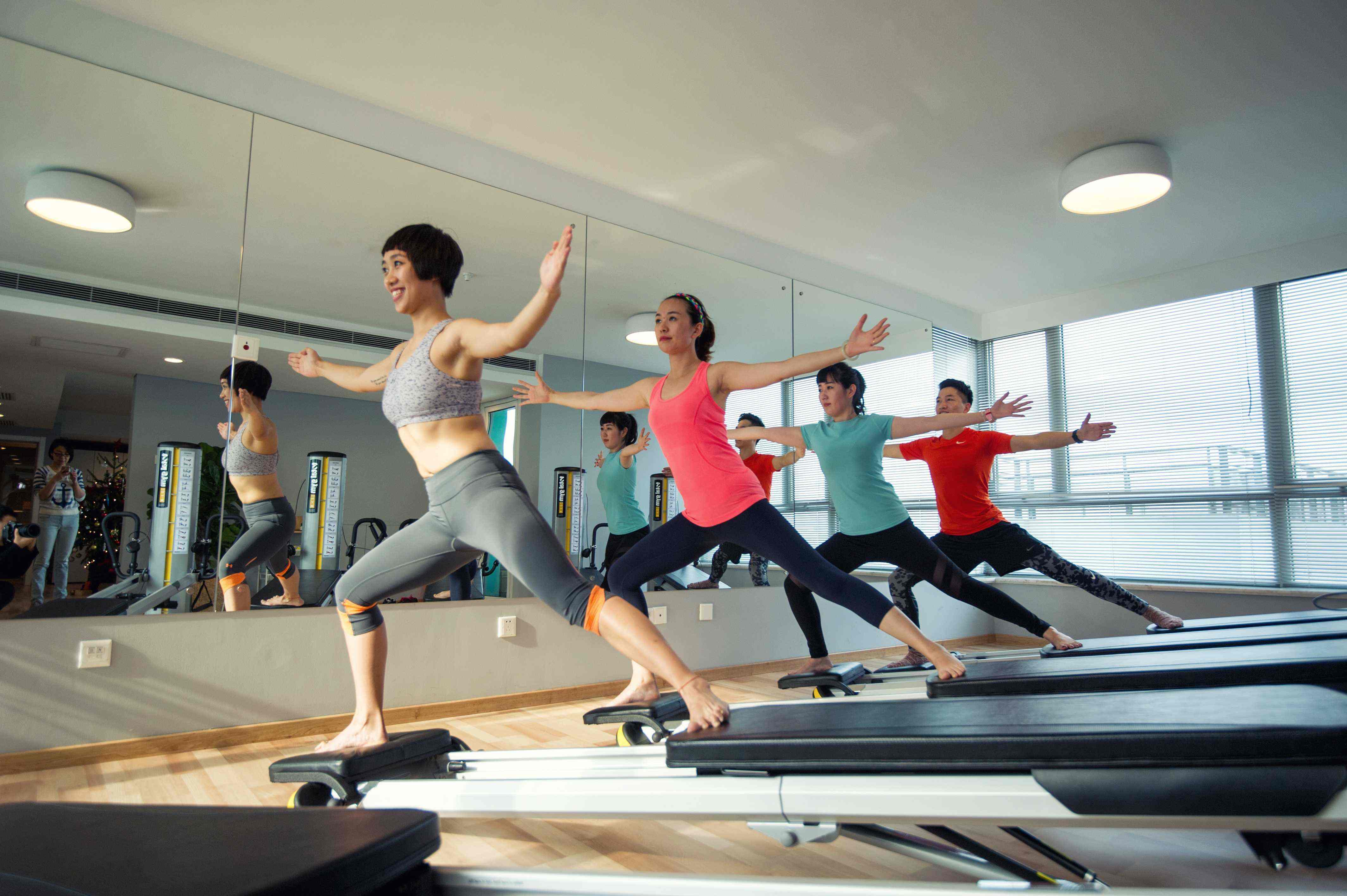
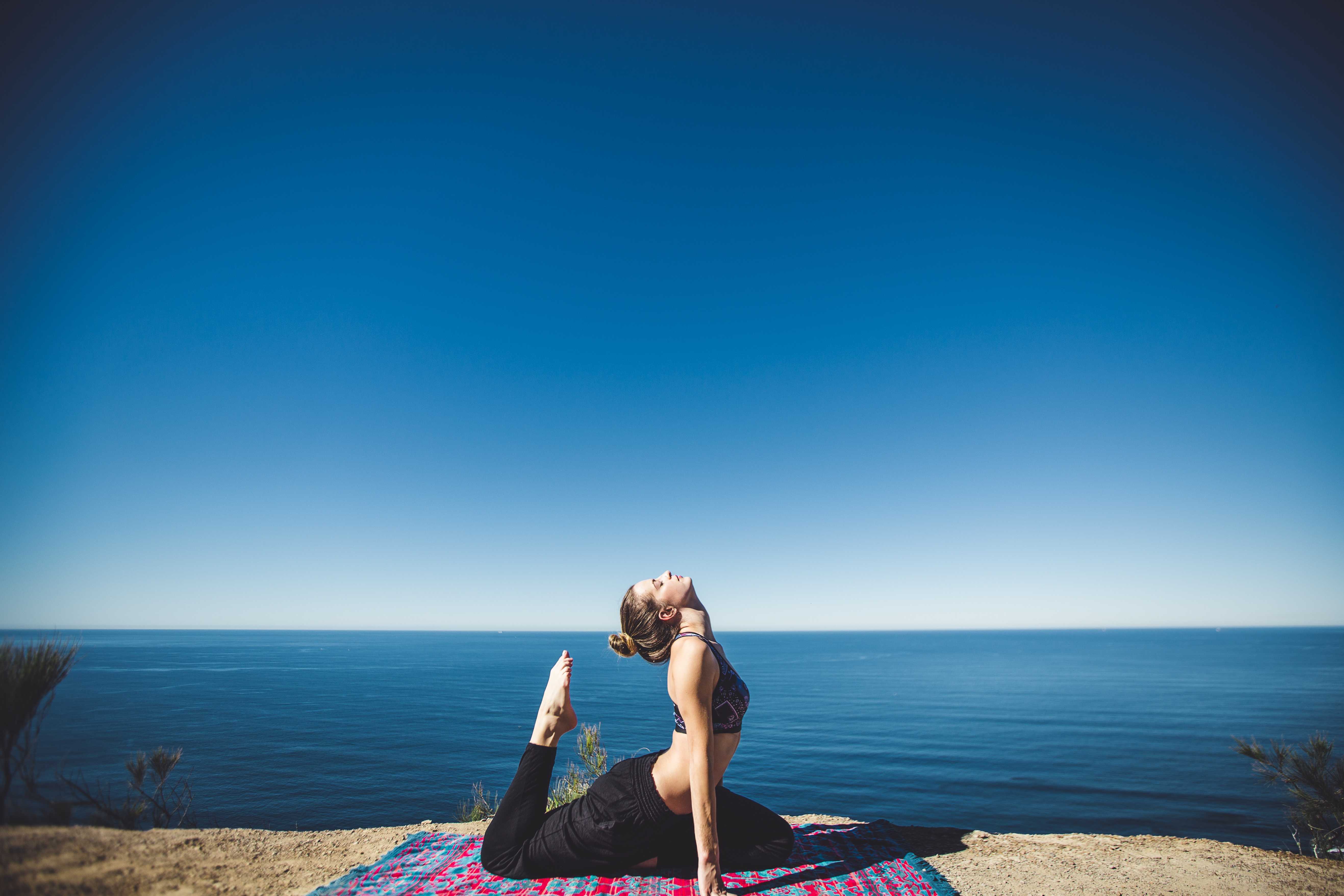
$ 30
Florence Pugh
Group Lessons

7:00 to 8:00 am

25 Peoples
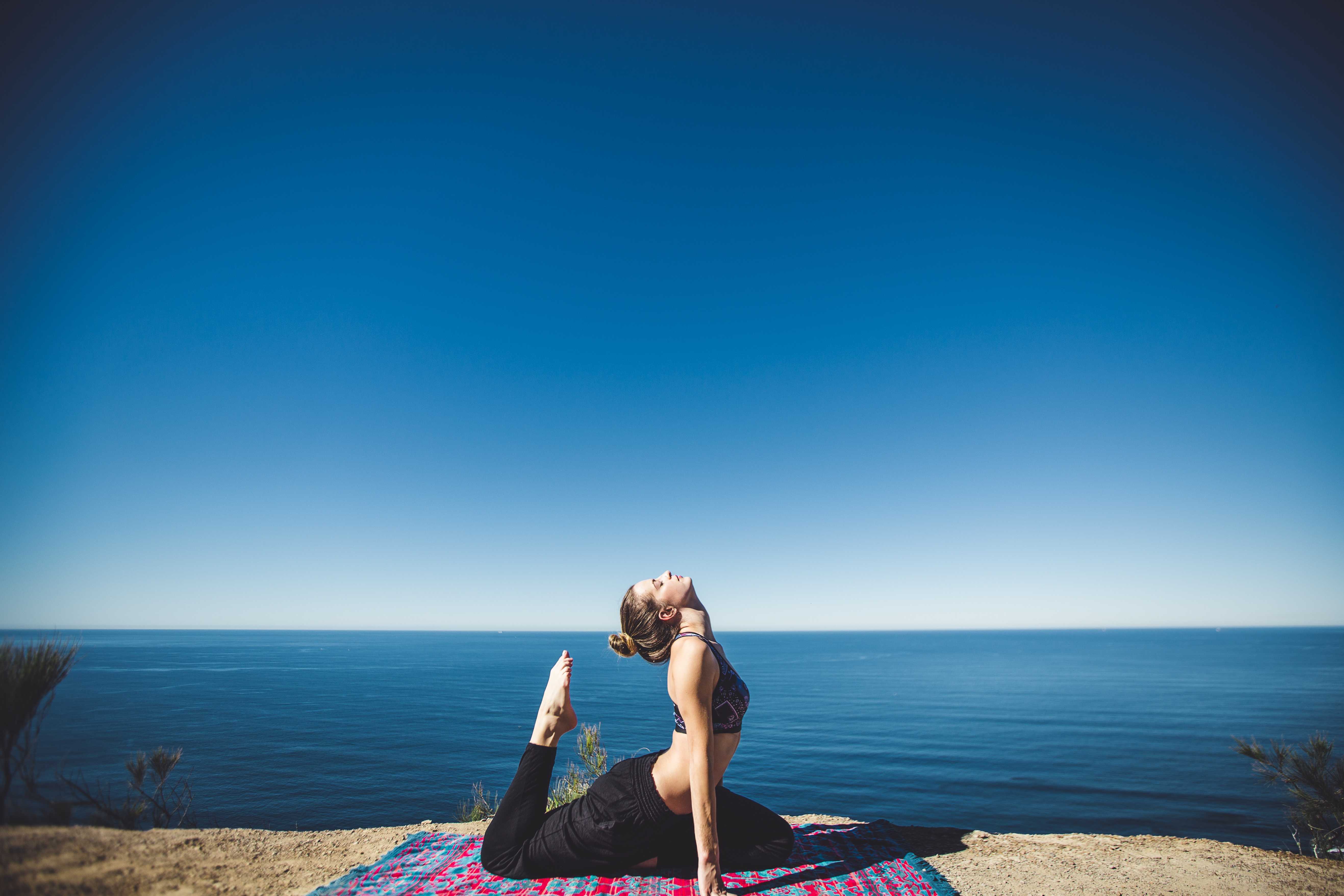
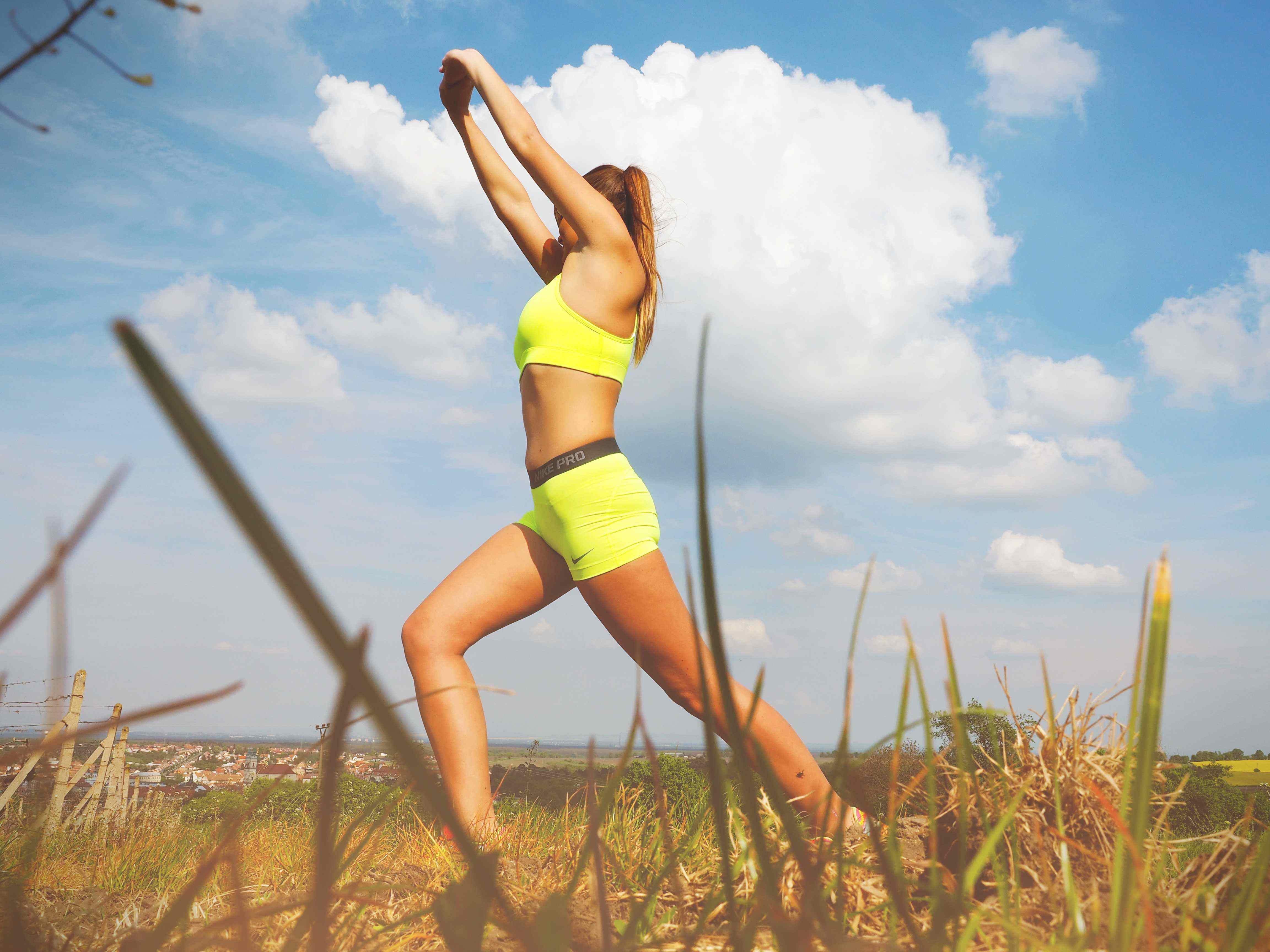
$ 30
Florence Pugh
Group Lessons

7:00 to 8:00 am

25 Peoples
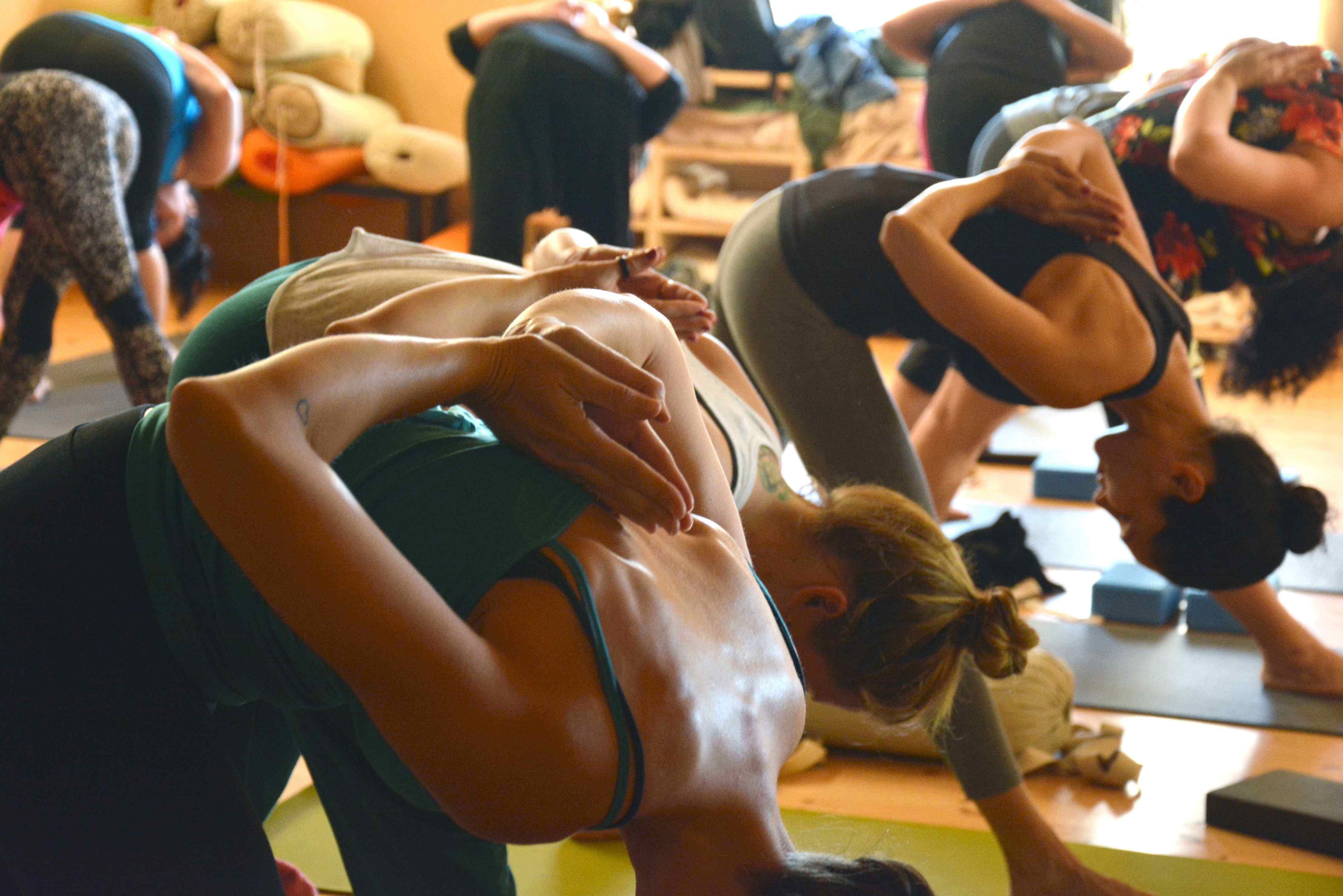
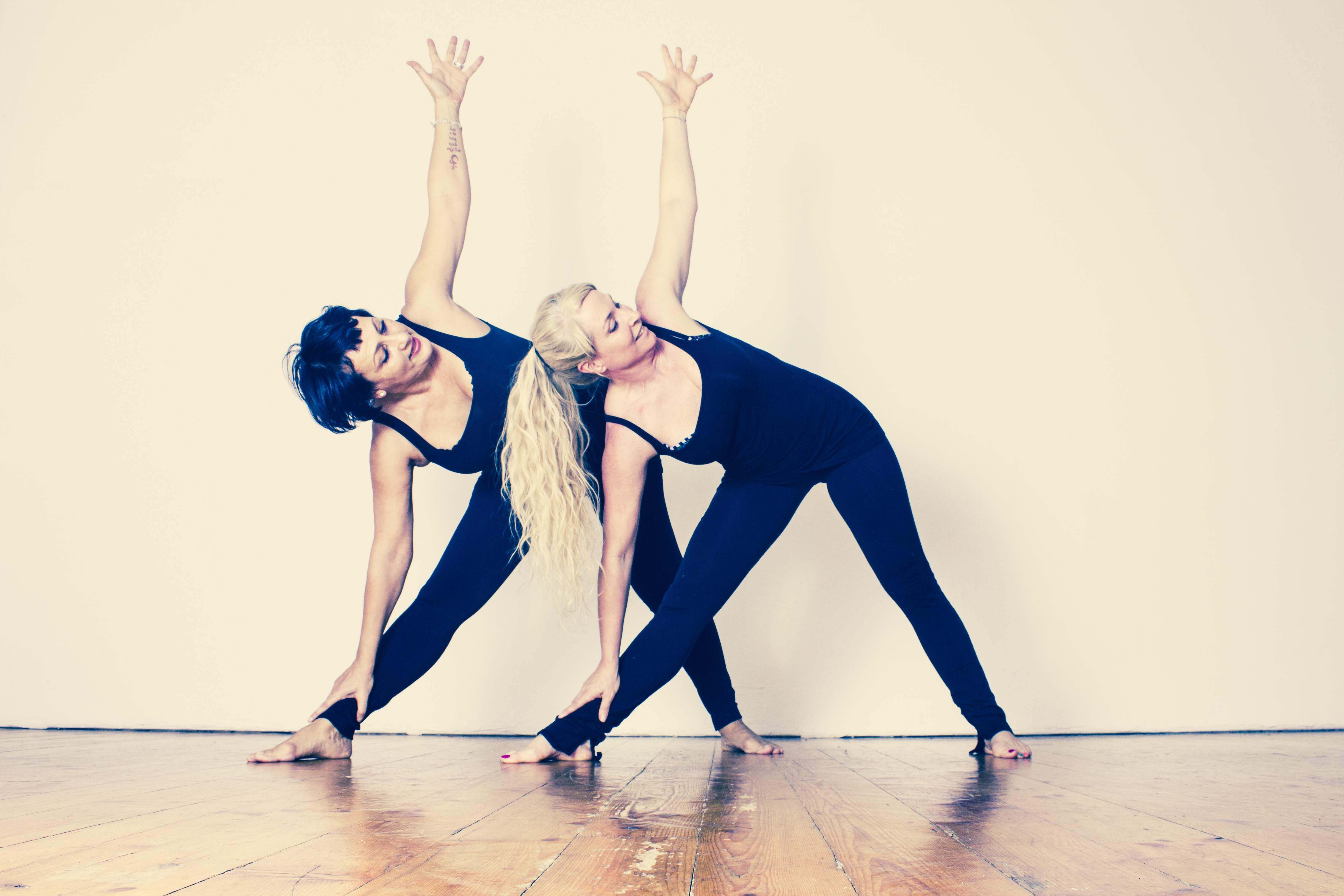
$ 30
Florence Pugh
Group Lessons

7:00 to 8:00 am

25 Peoples
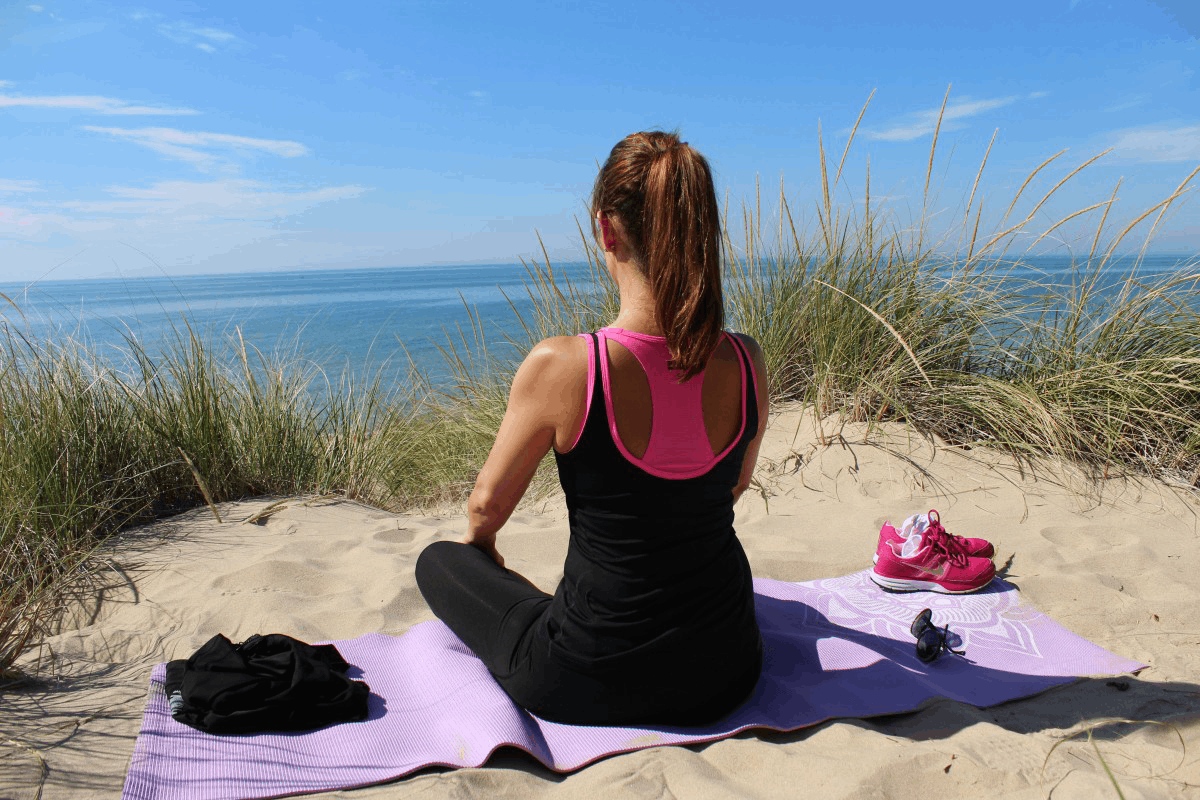
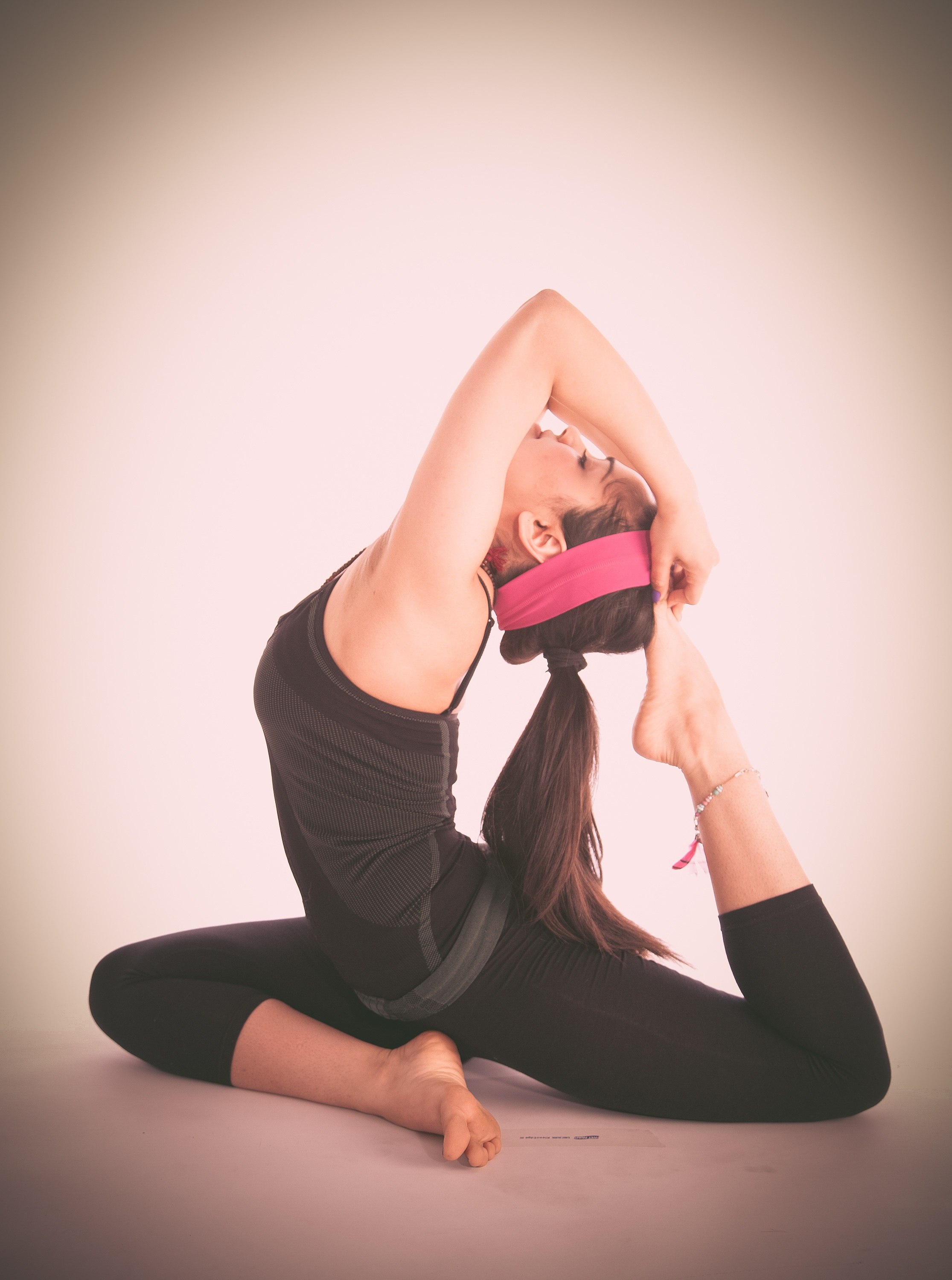
$ 30
Florence Pugh
Group Lessons

7:00 to 8:00 am

25 Peoples